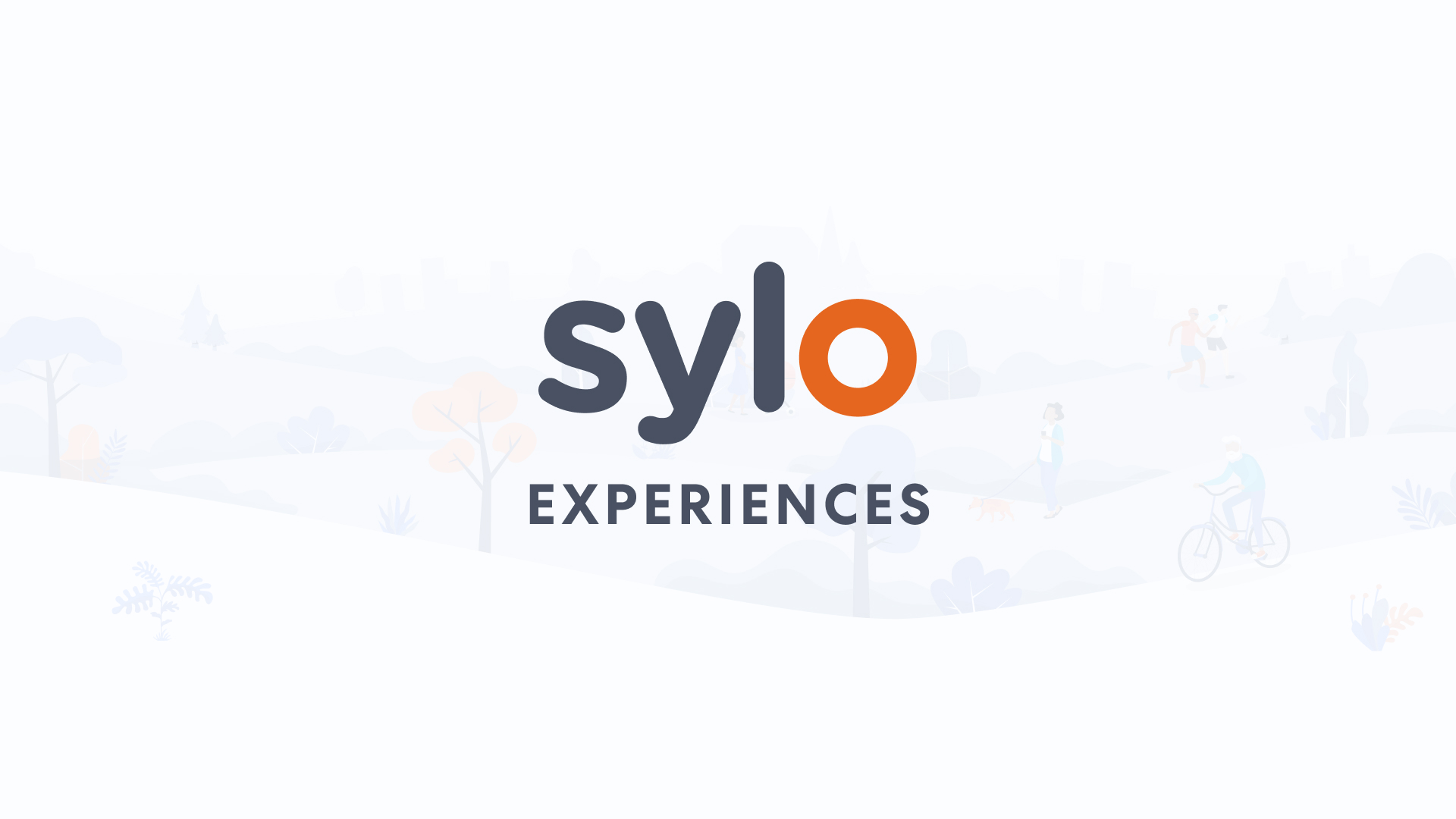
Sylo Experiences are custom built to enhance the Sylo Smart Wallet.
The framework is based on React Native so it should be just like building any other React Native application. Using the Sylo Experiences SDK means you can build your app and release it as a stand alone app or you can release it as an experience in Sylo. The advantage of releasing a Sylo Experience means that you get access to the existing Sylo user base, you don’t need to worry about registration and other things like payments are made super simple with the Sylo App API.
Getting Started
Installing dependencies
Depending on your platform you will need to install some dependencies before you get started.
- Node
- Watchman (MacOS)
- XCode (MacOS)
- Android Studio
- JDK
- The React Native CLI
Once you have that setup you should install the Sylo Experiences CLI.
npm i -g @sylo/connected-app-cli
Creating your project
Using the CLI we can create a new project from a template.
sylo-connected-app init [project-name]
Alternatively if you have an existing React Native project check that it is compatible and then you can publish.
Running your project
This will run your project as a stand alone app.
Android:
react-native run-android
iOS:
react-native run-ios
Once you have it running you should see something like this:
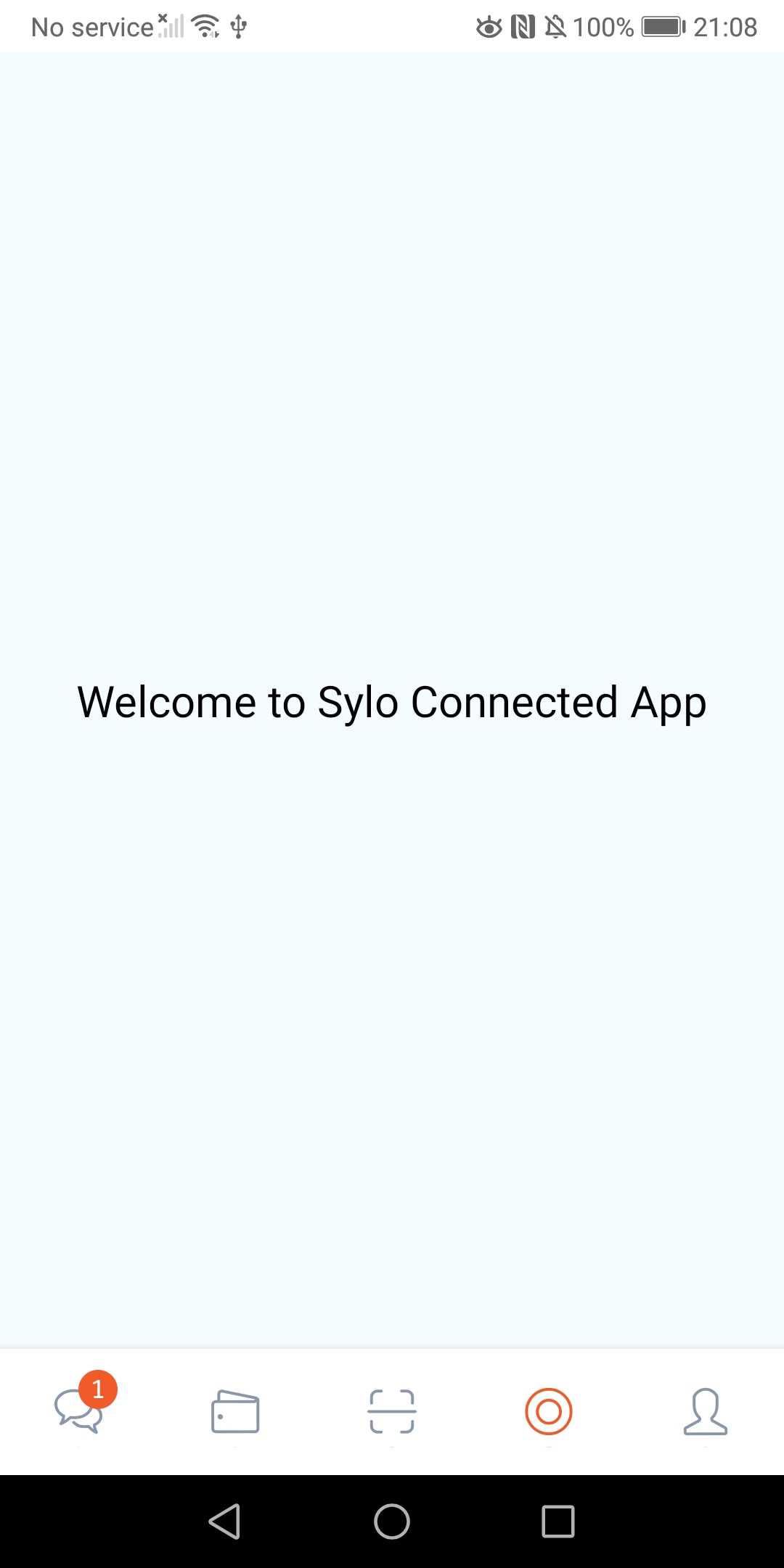
Build your experience
You’re away! You can now build your experience.
Here are some useful resources:
Further info
If you run into any issues or want to know more you can follow “React Native CLI Qickstart” in the React Native getting started guide.
Publishing
Now that you’ve built your experience you probably want to get it in the hands of Sylo users.
You can publish your experience to test it out in Sylo and share the experience via QR code.
sylo-connected-app publish
You will be prompted for a version which will be automatically incremented patches unless you specify it.
Once this has completed you should see a QR code, you can use this QR code to get your experience in Sylo.
Getting your experience in Sylo
You can either scan your QR code with the scanner in Sylo or you can enable developer mode in order for get more information
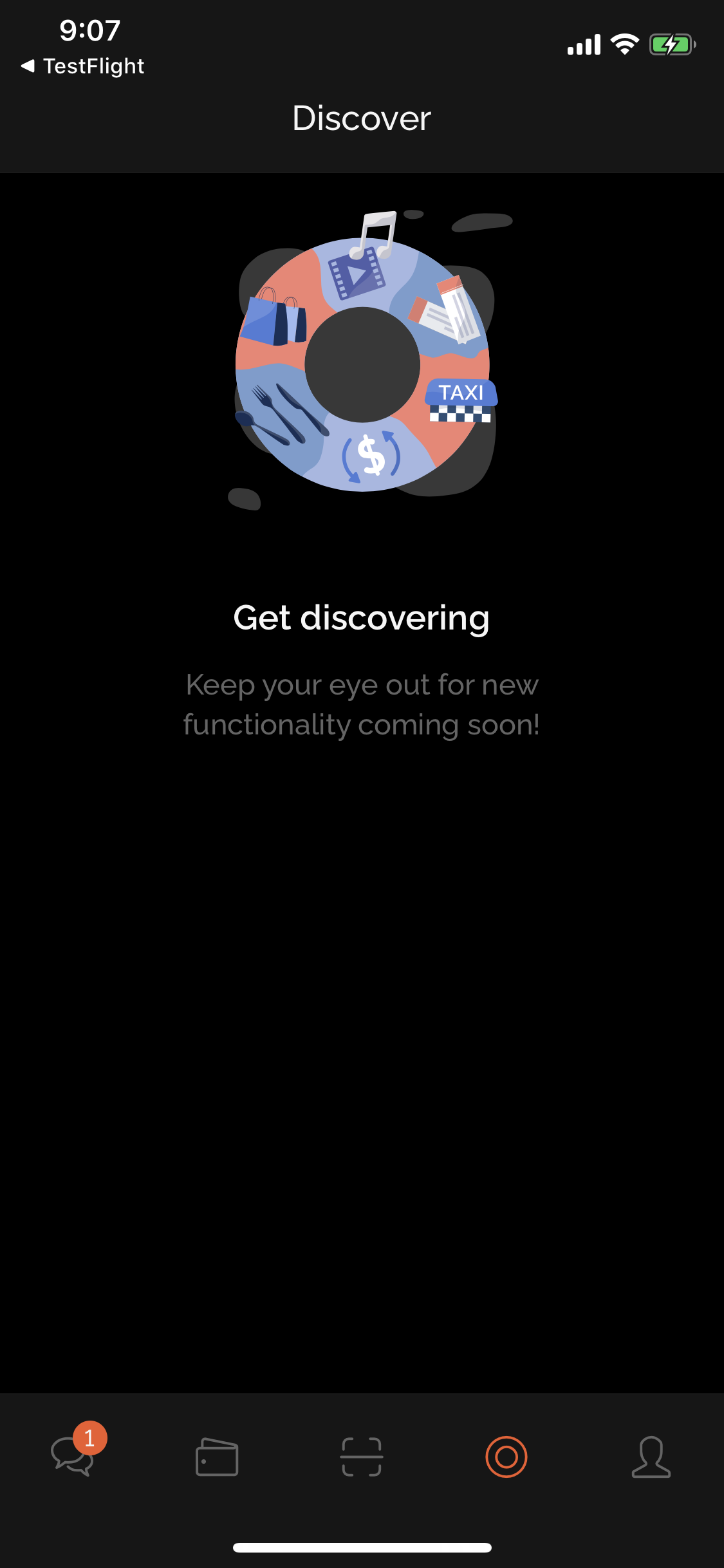
1. Navigate to the discover tab and press the header text 10 times
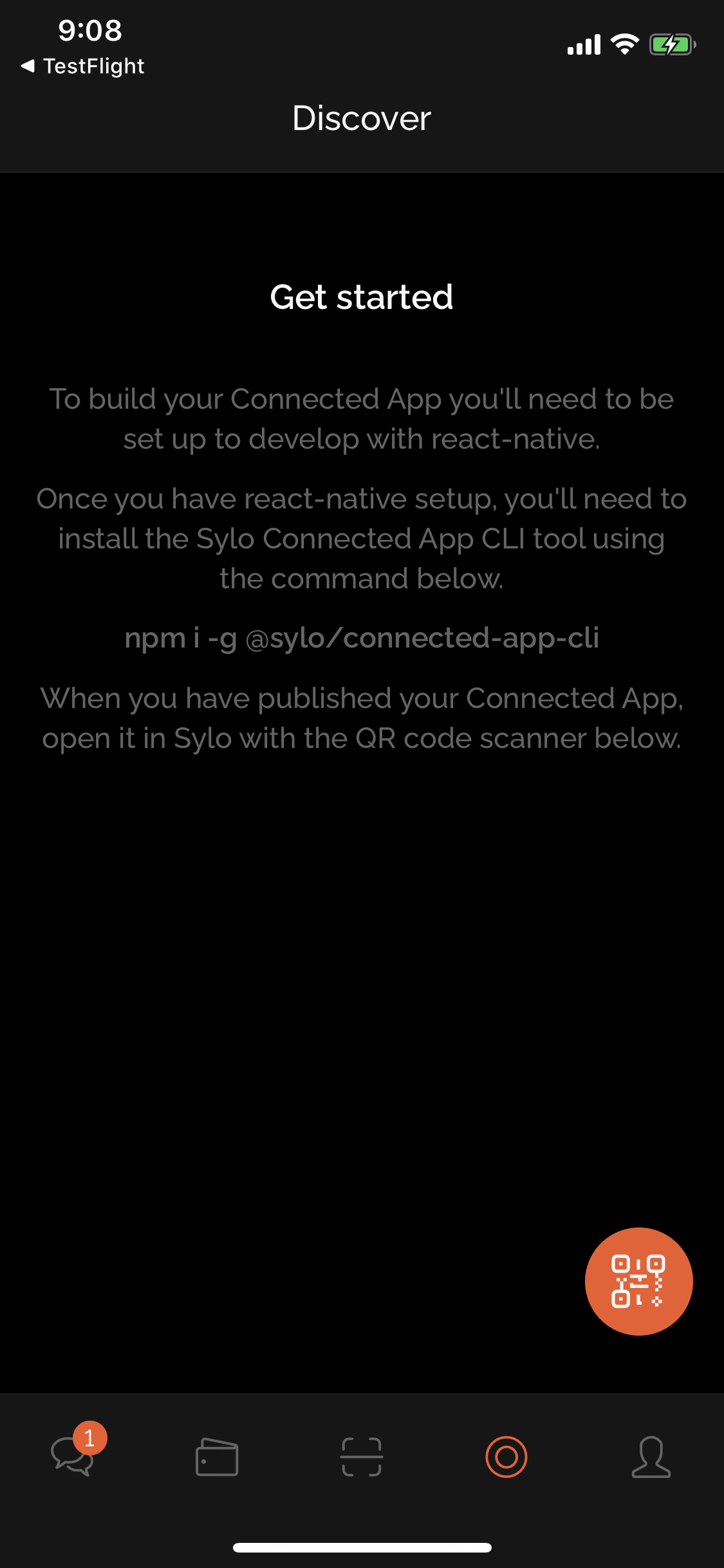
2. If that's successful you should see this screen
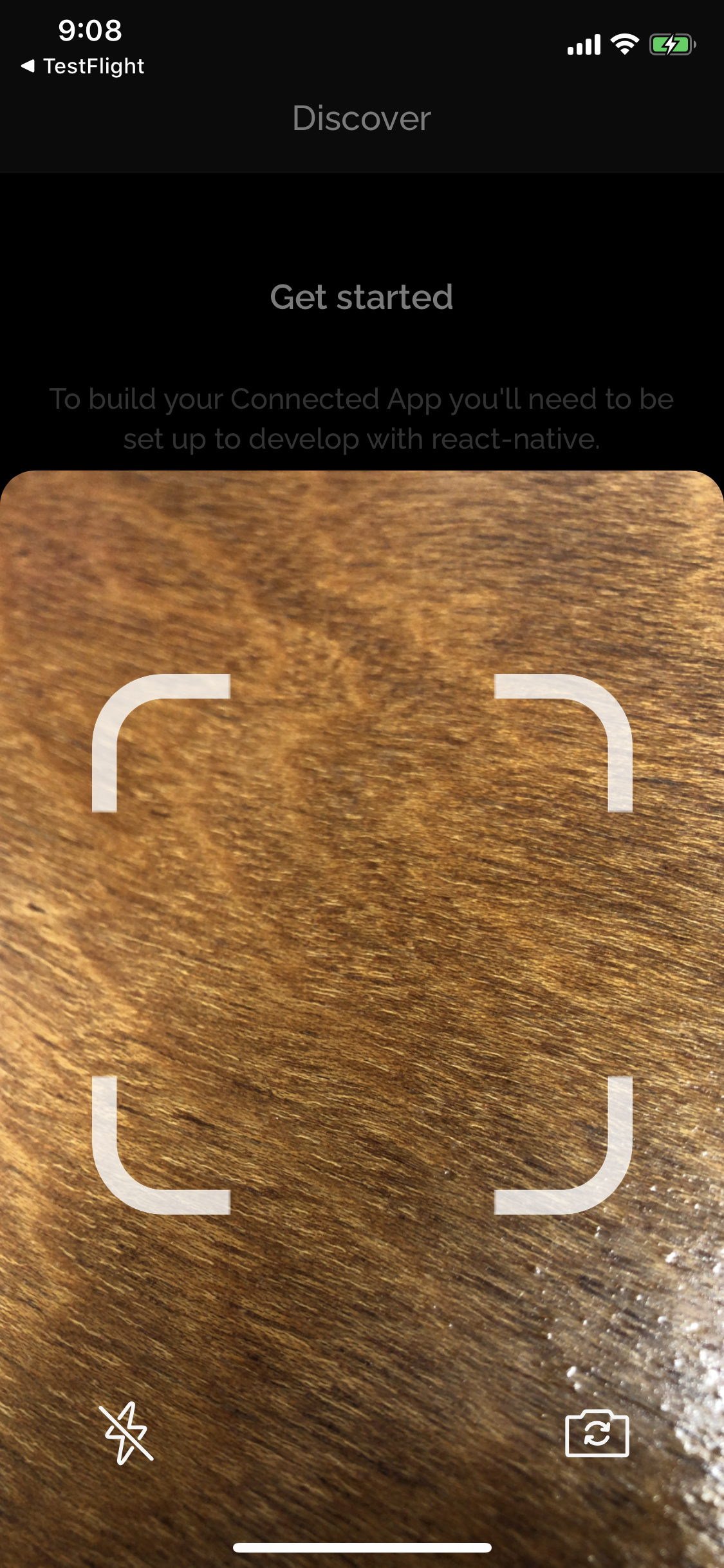
3. Press the scan button down the bottom and scan the QR code for your experience
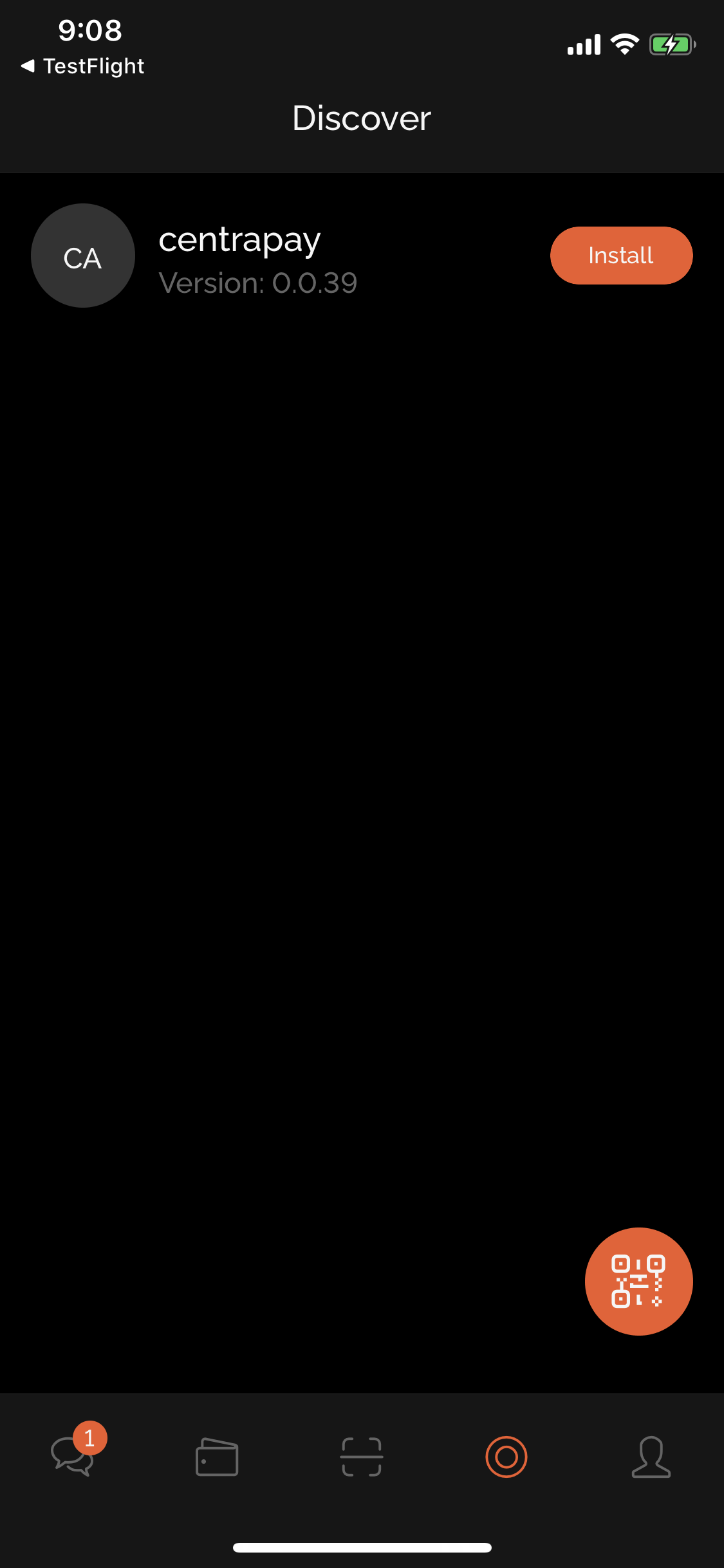
4. Press the install button for your experience
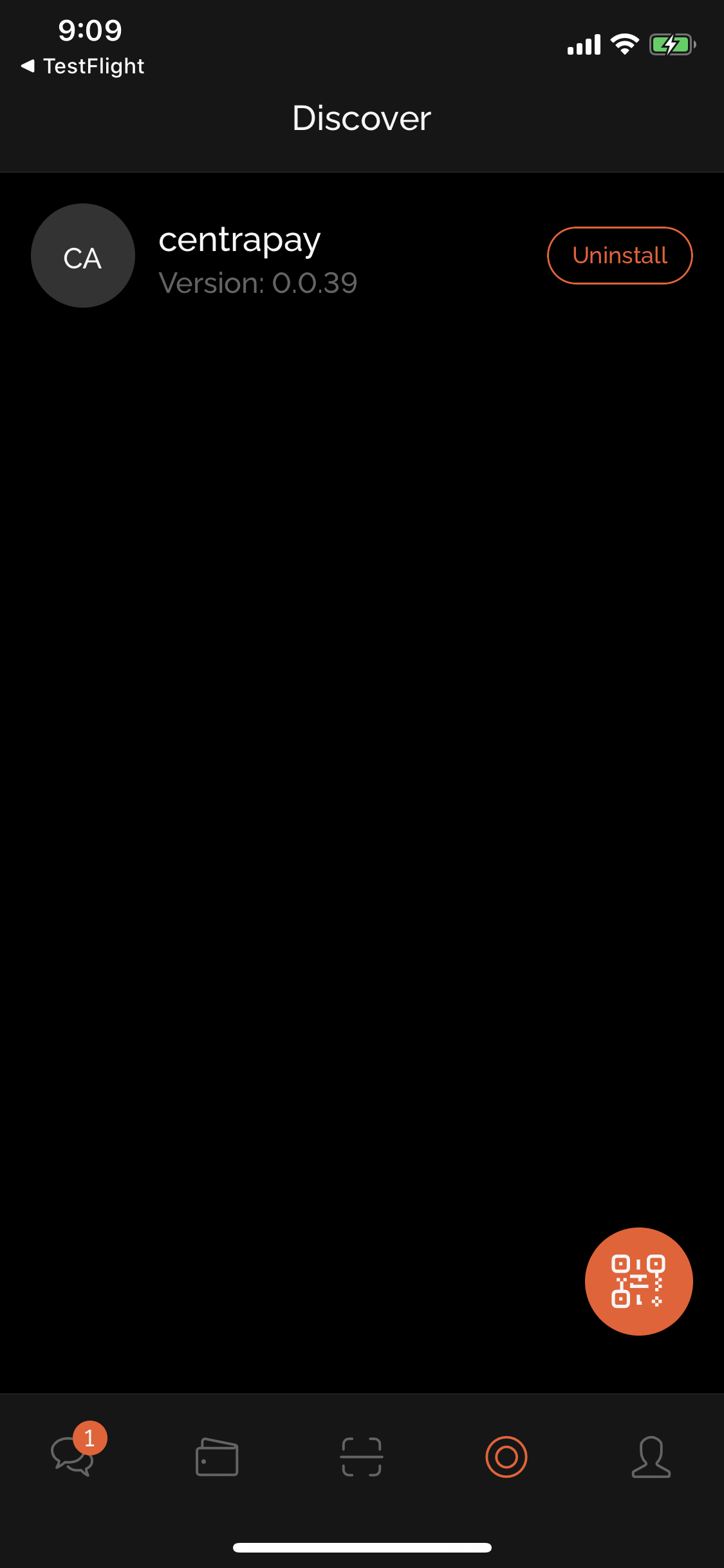
5. Press on your experience and you're away
Releasing Updates
As of now there is no update mechanism for experiences, in order to update you will have to publish a new version and distribute the new QR Code generated by it.
API Reference
The Sylo App API provides the ability to interact with the rest of the Sylo app, it gives you access to Bitcoin, Ethereum and Cennznet blockchains. It is only available when your experience is running within Sylo.
Usage
import { Api } from '@sylo/app-api'
const info = await Api.getInfo()
API
All of these functions will throw an error if they are called outside of the Sylo app
getInfo
Gets config info from Sylo
Api.getInfo(): Promise<{
ifpsEndpoint,
cennznetEndpoint,
syloVersion,
theme,
currency,
language
}>
getAccount
Gets public info on the account logged into sylo. Public key is a base64 encoded string
Api.getAccount(): Promise<{ publicKey, accountId }>
createBitcoinTx
Requests Sylo to create a bitcoin TX to an address for a specified amount. If successful, returns the transaction hash
Api.createBitcoinTx(
toAddress,
amount
): Promise<string>
Ethereum Provider
Sylo has implemented EIP-1193 this means you can use Web3.js or Ethers.js with an injected connector in the same way that Metamask works.
To set this up you need to add the following code before any other ethereum related imports
import { Api as SyloApi } from '@sylo/app-api'
window.ethereum = SyloApi.ethereumProvider
cennznetSigner
Sets the signer for the Cennznet Api. This means any Tx that wants to be signed will prompt the user in Sylo to sign the transaction.
import { Api as CennznetApi } from '@cennznet/api'
import { Api } from '@sylo/app-api'
api = await CennznetApi.create({
provider: 'wss://rimu.unfrastructure.io/public/ws'
})
api.setSigner(Api.cennznetSigner)
generateDoughnut
Sends a Doughnut Json Object to Sylo to be generated and signed by the account in Sylo. The parameters that are of type Uint8Array
can also be base64 encoded strings here.
This will prompt the user to approve the generation. If successful the doughnut will be a uint8array.
Api.generateDoughnut(doughnutRequest): Promise<Uint8Array>
If the user cancels the request then the promise is rejected with new Error("Request canceled")
Compatibility
In order for your project to remain compatible with Sylo Experiences there are certain requirements.
Dependencies
Any dependencies you add must be purely JS, anything that includes native code will not work. There are exceptions to this though, Sylo includes the native code for some dependencies with native modules. You can add them like you would like any other dependency. The exceptions are:
- react-native-camera@3.0.0
- react-native-randombytes@3.5.3
- react-native-reanimated@^1.1.0
- react-native-sodium
- react-native-svg@^9.4.0
- react-native-vector-icons@^6.6.0
- react-native-webrtc
React Native
React Native versions must be the same as what Sylo uses. Because Sylo is still maintained this changes from time to time, when it does change developers will be given plenty of warning when it is going to change so that experiences can be updated accordingly.
The current version is 0.61.2
Example Experiences
Future Development
APP API
The APP API is currently focused around being able to use the Sylo wallet to interact with blockchains, in the future you will be able to interact with the communication aspects of Sylo. Creating groups, sending messages and other interactions will be possible, this will be a privacy focused API.
Deep linking
Extending OS level deep linking to work within Sylo, thie will mean experiences can link to other experiences, share specific parts of your experience (e.g. a shop item) or open when QR codes are scanned in the Sylo scanner.
Custom messages
With deep linking comes the ability to share deep links, experiences will be able to create custom messages that can be shared to Sylo groups. Custom messages can create a window into your experience, these messages can be interactive, show live data and have an appearance built to your needs, even users without your experience can view these messages.
Wallet integration
- Associate your app with tokens you can interact with
- Resolve addresses in transactions
Distribution
We would like to make distribution of Sylo experiences easier, we plan to add a few features to make this easier.
- Bundle signing, there is nothing stopping experiences from having the same id currently, this means experiences can imitate other experiences, with bundle signing experiences will be verified
- Sharing experiences within Sylo, once people find a good experience they will be able to send that experience to their friends
- Updating, experiences need to be updatable.
- Experience meta data, we plan to extend this to include things like icons and developer info
Tooling
- Being able to better test the Sylo App API, currently you have to publish the app to test it out
- Error reporting and debugging in Sylo. Right now if apps are crashing in the wild developers have no visibility on this and what little visibility there is is very hard to figure out where issues are coming from
Other
We are open to expanding on the native dependencies that are available to developers in order to create better experiences